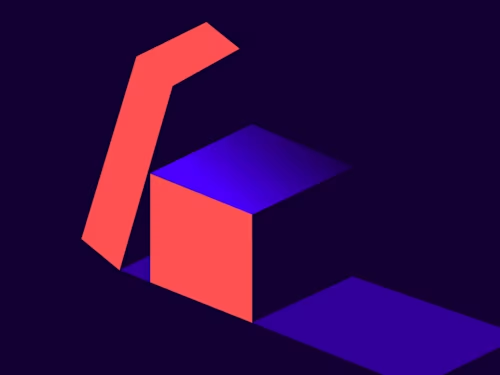
How to fix duplicate tabs in Docusign: Anchor vs. custom tabs
It's possible to inadvertently place duplicate tabs in a document when you add envelope-level or document-level tabs that match custom tabs applied at the account level. See how to troubleshoot and fix this issue.
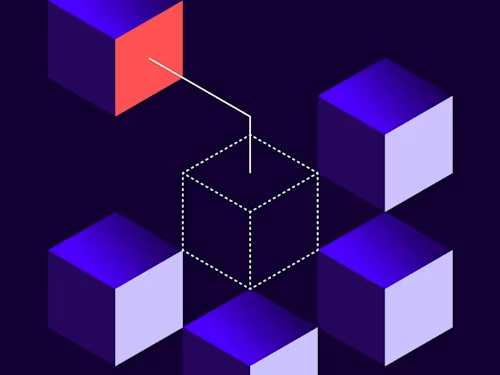
If you’ve configured custom tabs with auto anchors at the account level and are also passing anchor tags through your code, you might encounter duplicate tabs: for example, seeing four tabs instead of the expected two. In this post, I’ll explore why this happens, how these features work, and show you how to resolve it.
Understanding Docusign Custom Tabs: Manual Placement vs. Auto-Place with {r} Marker
Before diving into debugging the issue, it's essential to understand the key differences between two powerful Docusign features (account-level custom tabs that exist are regular tabs created for the account, while account-level custom tabs with the {r} marker are special tabs that automatically place themselves in the layout) and how they are helpful.
When adding fields in Docusign, you might come across auto-place with custom tags and anchor tabs. Both use anchor text to position fields, but they work differently. Auto-place with custom tags uses predefined anchor text to automatically position fields and can include account-level custom tags or {r} markers, which link fields to specific recipient roles, ensuring the right person receives the correct field. This method is commonly used in Docusign for Salesforce, where recipient roles dynamically determine tab placement. On the other hand, Standard Anchor Tabs are built-in Docusign tabs, such as signature, date, or initials, placed using anchor text. Unlike {r}-based custom tags, they do not adjust dynamically to recipient roles. Their placement is fixed, but you can refine their position using offsets.
The key takeaway is that Auto-Place works with both standard and custom tabs by placing fields based on anchor text. Custom tags with {r} adapt to recipient roles, while standard anchor tabs remain static. Additionally, tabs can be stored at the account level for reuse or added directly at the envelope level as needed.
How can this happen?
As mentioned earlier, if you have created document custom tabs with automatic anchor taggings at the account level, then when you create an envelope through the SDK, Postman, or web interface, additional anchor tabs with the same auto-place text (for example, "Sign Here") may be generated. This results in duplicates, causing multiple signing prompts at the same location on the document, as these tabs overlap in the same position.
Additionally, the same issue can occur with anchor tabs when their anchor data population scope is set to the envelope level instead of the document level while creating envelopes from templates. This can lead to duplicated signing tabs across documents.
The solution: How to fix duplicate tabs
To resolve this kind of issue, the recommended workaround is to remove the custom tabs that were manually created with the same anchor text as one of the fields. Alternatively, you can also remove the anchors in your createEnvelope API request, which should resolve the issue.
To remove the custom tabs in your account through the API, first call the CustomTabs: list endpoint to get a list of your account-level custom tabs. This will return a JSON object containing each tab’s properties, including the customTabId.
Call:
GET
/restapi/v2.1/accounts/{account ID}/tab_definitions?custom_tab_only=true
Response:
{
"tabs": [
{
"font": "lucidaconsole",
"bold": "false",
"italic": "false",
"underline": "false",
"fontColor": "black",
"fontSize": "size9",
"anchor": "\\vlcSignDate{r}\\",
"height": "0",
"width": "0",
"customTabId": "795f51e1-xxxx-xxxx-xxxx-70433777cf7f",
"initialValue": "",
"disableAutoSize": "false",
"concealValueOnDocument": "false",
"locked": "false",
"required": "false",
"shared": "true",
"collaborative": "false",
"requireInitialOnSharedChange": "false",
"requireAll": "false",
"tabLabel": "Date Signed",
"maximumLength": "",
"name": "",
"type": "text",
"validationMessage": "",
"validationPattern": "",
"lastModifiedByUserId": "6d028681-xxxx-xxxx-xxxx-a59bbb5cb34a",
"lastModifiedByDisplayName": "saitest1 dandamudi2",
"lastModified": "2025-01-19T23:34:45.6900000Z",
"createdByUserId": "6d028681-xxxx-xxxx-xxxx-a59bbb5cb34a",
"createdByDisplayName": "saitest1 dandamudi2",
"editable": "true"
},
]
}
Delete the conflicting custom field
You can remove account-level custom tabs with the following API call:
DEL
/restapi/v2.1/accounts/{accountId}/tab_definitions/{customTabId}
Here’s an example request body showing how to specify the account-level tabs you want to remove:
static string SendDocuSignEnvelope()
{
Title titleTab1 = new Title
{
AnchorIgnoreIfNotPresent = "true",
AnchorString = "\vlcTitle1\",
Name = "titleTabs1",
RecipientId = "1"
};
List<Title> titleTabs1 = new List<Title> {titleTab1};
FullName fullNameTab1 = new FullName
{
AnchorIgnoreIfNotPresent = "true",
AnchorString = "\vlcFullName1\",
Name = "fullNameTabs1",
RecipientId = "1"
};
List<FullName> fullNameTabs1 = new List<FullName> {fullNameTab1};
}
Another workaround to resolve the duplicate tabs is to use explicitly placed tabs at the document level, rather than anchor strings. Here’s an example of what it looks like to specify manually placed tabs in the request body of the createEnvelope API call:
SignHere signHereTab1 = new SignHere
{
DocumentId = "1",
PageNumber = "1",
RecipientId = "1",
ScaleValue = ".7",
XPosition = "134",
YPosition = "144"
};
List<SignHere> signHereTabs1 = new List<SignHere> {signHereTab1};
Tabs tabs1 = new Tabs
{
SignHereTabs = signHereTabs1
};
Finally, here’s an example of how you specify document-level anchor tabs in your createEnvelope request body:
private static Document CreateDocument(string documentId, string name)
{
return new Document
{
DocumentBase64 = "",
DocumentId = documentId,
Name = name
};
}
private static Signer CreateSigner(string email, string name, string recipientId, List<Text> textTabs)
{
return new Signer
{
Email = email,
Name = name,
RecipientId = recipientId,
Tabs = new Tabs { TextTabs = textTabs }
};
}
private static CompositeTemplate CreateCompositeTemplate(string templateId, Document document, string sequence, List<Signer> signers)
{
return new CompositeTemplate
{
CompositeTemplateId = templateId,
Document = document,
InlineTemplates = new List<InlineTemplate>
{
new InlineTemplate
{
Sequence = 1,
Recipients = new Recipients { Signers = signers }
}
}
};
}
public static string SendDocuSignEnvelope()
{
var document = CreateDocument("1", "Text.pdf");
var textTab = new Text
{
AnchorString = "Anchor",
Height = "20",
Width = "120",
XPosition = "130",
YPosition = "220"
};
var signer = CreateSigner("Test.Demo1@example.com", "Sai Text", "1", new List<Text> { textTab });
var compositeTemplate = CreateCompositeTemplate("1", document, "1", new List<Signer> { signer });
var envelopeDefinition = new EnvelopeDefinition
{
CompositeTemplates = new List<CompositeTemplate> { compositeTemplate },
EmailSubject = "Please sign this document",
Status = "sent"
};
return "Envelope Sent"; // Replace with actual envelope sending logic
}
Alternatively, if you’d prefer to delete these custom tabs from the UI, then please follow the Docusign Support instructions.
Best practices to avoid duplication
To avoid overlapping tabs and alignment issues when using auto-place with custom tags, make sure that each field has a unique anchor text. Duplicate tabs can occur if the same anchor string is used across multiple fields. This issue may also arise if the anchor data population scope is set at the envelope level rather than the document level when creating envelopes from templates. To prevent this, use unique anchor strings for each field and always specify document details in the code to set the anchor tab population for a specific document, rather than across the entire envelope in the Docusign Web UI.
Additionally, if the Automatically replicate information in fields with the same data label option is enabled (to enable this feature, please reach out to DS Support) for all documents in an envelope, instead of on an individual document, the anchor tabs will apply to all the anchor strings across the envelope, affecting all documents and pages, which can lead to duplication.
Wrapping up
By understanding the differences between anchor and custom tabs at the account, envelope, and document level and their interplay, you can prevent the frustration of duplicate tabs and ensure a seamless signing experience for recipients. You can resolve this issue by cleaning up custom tabs at the account level or by adjusting your payload: removing anchors or setting up data population scope at the document level instead of the envelope level and specifying document IDs in code, as I’ve shown.
Additional resources
Sai Dandamudi has been with Docusign since 2022. Her work is focused in helping developers resolve issues they encounter when developing applications using Docusign APIs and advising developers on how to integrate with Docusign APIs and SDKs by consulting on best practices and providing code examples. You can reach out to Sai through Linkedin.
Related posts
- Developer Support ArticlesPublished Sep 21, 2021
- Developer Support ArticlesPublished Feb 26, 2020
From the Trenches: Tabs and Custom Fields
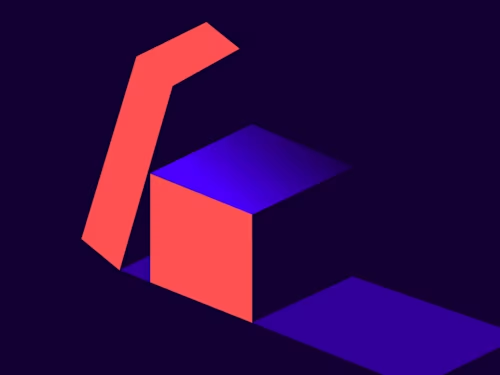
From the Trenches: Anchor string throwing errors
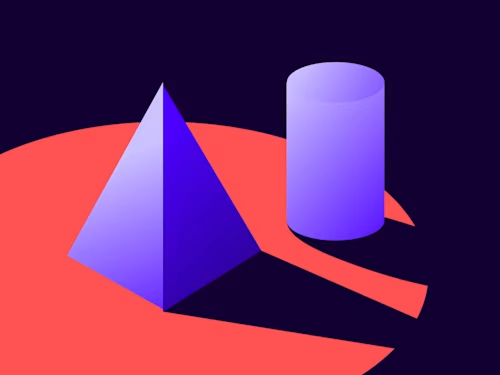
From the Trenches: Tabs and Custom Fields
Discover what's new with Docusign IAM or start with eSignature for free
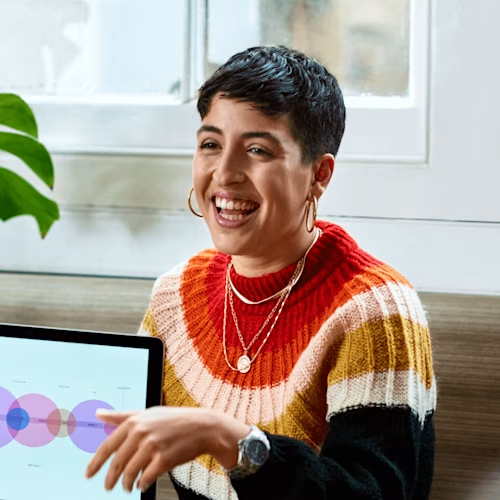