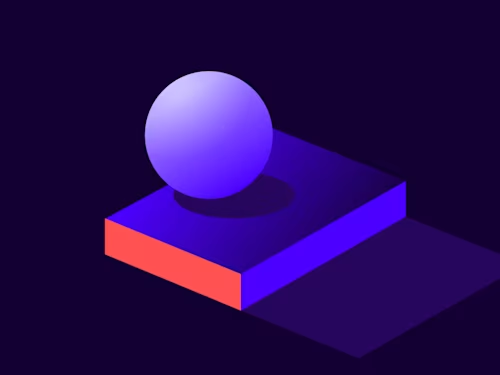
Common API Tasks🐈: Download a document that is part of an eSignature template
Inbar Gazit shows you how to download a document from a template using the eSignature REST API.
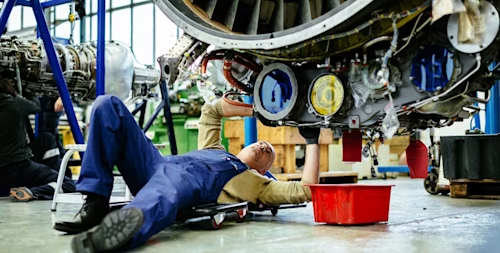
Welcome to a splendid new edition of the CAT🐈 (Common API Tasks) blog series. The CAT blogs provide all you need to complete small, specific, SDK-supported tasks using one of our APIs. You can find all articles in this series on the Docusign Developer Blog.
Have you ever used an eSignature template? If so, you know that it includes one or more documents. These documents represent the visual that will be used for the eSignature process. They will be copied over to any envelope created from this template, which means that these documents are a critical part of the business process. This is why you may need to obtain one of these documents from the template itself rather than from an envelope. For example, you may want to modify a document, but you may not have the original handy. Or you may want to compare the documents in two different templates. Your system may just want to show this document to the end user before they pick which template they are going to use to create an envelope. This is what I’ll be showing you in this latest edition of the blog series.
The code snippets in here are short and simple. What you need to have in order to run them is a valid auth token (that is needed for any API call), the accountID (GUID) for the specific Docusign account, the templateID for the specific template you’re using (you can find that on the Docusign web app, or by making another API calls to get the list of all the templates), and finally, the documentId, as you may have more than one document in your template (I hardcoded “1” for these snippets). And here is how you get this done.
C#
var docuSignClient = new DocuSignClient(basePath);
// You will need to obtain an access token using your chosen authentication method
docuSignClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
TemplatesApi templatesApi = new TemplatesApi(docuSignClient);
System.IO.Stream doc = templatesApi.getDocument(accountId, templateId, "1");
// The doc variable now contains the bytes of the PDF downloaded from Docusign, you can use this to store to disk or show to a web user
Java
Configuration config = new Configuration(new ApiClient(basePath));
// You will need to obtain an access token using your chosen authentication method
config.addDefaultHeader("Authorization", "Bearer " + accessToken);
TemplatesApi templatesApi = new TemplatesApi(config);
byte[] doc = templatesApi.GetDocument(accountId, templateId, "1");
// The doc variable now contains the bytes of the PDF downloaded from Docusign, you can use this to store to disk or show to a web user
Node.js
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
// You will need to obtain an access token using your chosen authentication method
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
let templatesApi = new TemplatesApi(dsApiClient);
let doc = templatesApi.GetDocument(accountId, templateId, '1');
// The doc variable now contains the bytes of the PDF downloaded from Docusign, you can use this to store to disk or show to a web user
PHP
$api_client = new \DocuSign\eSign\client\ApiClient($base_path);
$config = new \DocuSign\eSign\Model\Configuration($api_client);
# You will need to obtain an access token using your chosen authentication method
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
$templates_api = new \DocuSign\eSign\Api\EnvelopesApi($api_client);
$doc = $templates_api->getDocument($account_id, '1', $template_id);
# The $doc variable now contains the bytes of the PDF downloaded from Docusign, you can use this to store to disk or show to a web user
Python
api_client = ApiClient()
# You will need to obtain an access token using your chosen authentication method
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
templates_api = EnvelopesApi(api_client)
doc = templates_api.get_document(account_id=account_id,document_id='1',template_id=template_id);
# The doc variable now contains the bytes of the PDF downloaded from Docusign, you can use this to store to disk or show to a web user
Ruby
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
# You will need to obtain an access token using your chosen authentication method
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
templates_api = DocuSign_eSign::EnvelopesApi.new api_client
doc = templates_api.get_document account_id, '1', envelope_id
# The doc variable now contains the bytes of the PDF downloaded from Docusign, you can use this to store to disk or show to a web user
That’s all, folks! I hope you found it useful. If you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to email me. Until next time…
Additional resources
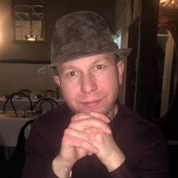
Inbar Gazit has been with Docusign since 2013 in various engineering roles. Since 2019 he has focused on developer content. Inbar works on code examples including the launchers, available on GitHub in eight languages, and helps build sample apps showcasing the various Docusign APIs. He is also active on StackOverflow, answering your questions. Inbar can be reached at inbar.gazit@docusign.com.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
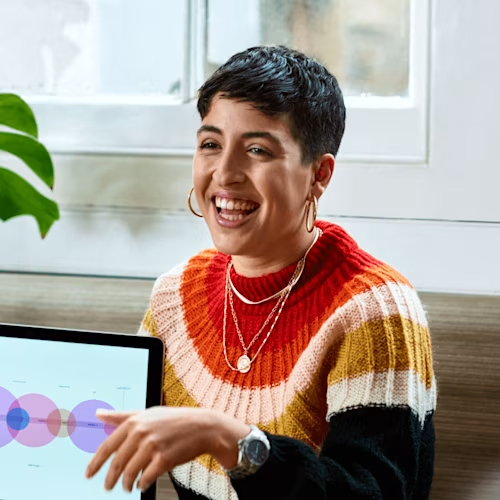