Common API Tasksš: Create a signing group
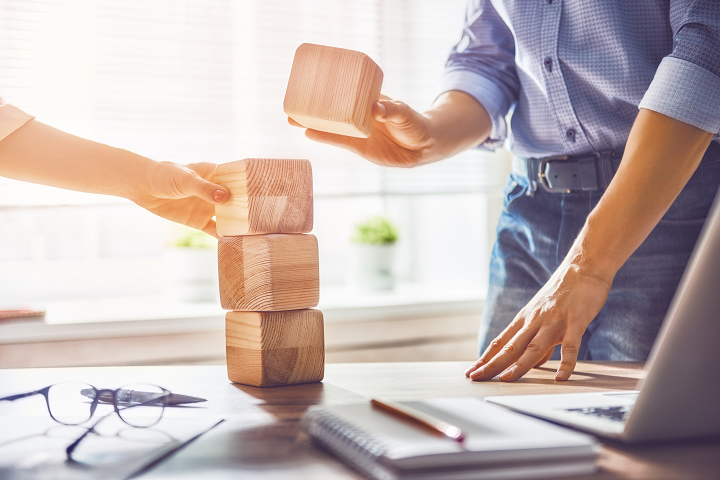
Welcome to the seventh issue of our Common API Tasks blog series. I hope by now you had a chance to read any of the six prior posts in the Common API Tasks series. This series is all about helping you, the developers using Docusign APIs, to complete popular tasks that are commonly used in applications. In this post, Iām going to talk about how you use our eSignature REST API to create a new signing group.Ā Ā
What are signing groups?
Imagine that you have a repetitive process: say, an invoice that has to be signed by one of the procuring managers in the company. To make things simple, letās assume you have only two such managers: Sally and Dan. Only one of them needs to sign the invoice. Sometimes Dan is out on vacation, sometimes Sally is busy. To whom do you send it? You donāt want to change your process each time based on Sallyās and Danās availability, as that would be inefficient.
The solution is to use Docusign signing groups. A signing group is a predefined set of recipients that can be added to any envelope in the same Docusign account in which it was created. Itās an account-wide feature that can be used by any user in the account; therefore, only account administrators can make changes to signing groups. To add a signing group from Docusign eSignature Admin, select Signing Groups; then click ADD SIGNING GROUP:

Ā
This interface enables the Docusign administrators to manage their signing groups. However, this can also be done using the eSignature REST API. Here is how:
Ā
C#
// You would need to obtain an accessToken using your chosen auth flow
var apiClient = new ApiClient(basePath);
apiClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
SigningGroupsApi signingGroupsApi = new SigningGroupsApi(apiClient);
SigningGroup mySigningGroup = new SigningGroup();
mySigningGroup.GroupName = "Managers";
mySigningGroup.GroupType = "sharedSigningGroup"; // this value means it's a signing group
mySigningGroup.Users = new List<SigningGroupUser>();
// every user must have an email address and a user name
mySigningGroup.Users.Add(new SigningGroupUser { Email = "sally@example.com", UserName = "Sally Doe" });
mySigningGroup.Users.Add(new SigningGroupUser { Email = "dan@example.com", UserNameĀ = "Dan Dan" });
SigningGroupInformation signingGroupInfo = new SigningGroupInformation();
signingGroupInfo.Groups = new List<SigningGroup>();
signingGroupInfo.Groups.Add(mySigningGroup);
signingGroupsApi.CreateList(accountId, signingGroupInfo);
Java
// You would need to obtain an accessToken using your chosen auth flow
Configuration config = new Configuration(new ApiClient(basePath));
config.addDefaultHeader("Authorization", "Bearer " + accessToken);
SigningGroupsApi signingGroupsApi = new SigningGroupsApi(config);
SigningGroup mySigningGroup = new SigningGroup();
mySigningGroup.setGroupName("Managers");
mySigningGroup.setGroupType("sharedSigningGroup"); // this value means it's a signing group
// every user must have an email address and a user name
SigningGroupUser userItem1 = new SigningGroupUser();
userItem1.setEmail("sally@example.com");
userItem2.setUserName("Sally Doe");
SigningGroupUser userItem2 = new SigningGroupUser();
userItem1.setEmail("dan@example.com");
userItem2.setUserName("Dan Dan");
mySigningGroup.addUsersItem(userItem1);
mySigningGroup.addUsersItem(userItem2);
SigningGroupInformation signingGroupInfo = new SigningGroupInformation();
signingGroupInfo.addGroupsItem(mySigningGroup);
signingGroupsApi.createList(accountId, signingGroupInfo);
Node.js
// You would need to obtain an accessToken using your chosen auth flow
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
let signingGroupsApi = new docusign.SigningGroupsApi(dsApiClient);
let mySigningGroup = new docusign.SigningGroup();
mySigningGroup.groupName = 'Managers';
mySigningGroup.groupType = 'sharedSigningGroup'; // this value means it's a signing group
// every user must have an email address and a user name
let userItem1 = new docusign.SigningGroupUser();
userItem1.email= 'sally@example.com';
userItem2.userName = 'Sally Doe';
let userItem2 = new docusign.SigningGroupUser();
userItem1.email = 'dan@example.com';
userItem2.userName = 'Dan Dan';
let userItems = [];
userItems.push(userItem1);
userItems.push(userItem2);0
mySigningGroups.users = userItems;
let signingGroupInfo = new SigningGroupInformation();
let groups = [];
groups.push(mySigningGroup);
signingGroupInfo.groups = groups;
signingGroupsApi.createList(accountId, signingGroupInfo);
PHP
# You would need to obtain an accessToken using your chosen auth flow
$api_client = new \Docusign\eSign\client\ApiClient($base_path);
$config = new \Docusign\eSign\Model\Configuration($api_client);
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
$signing_group_api = new \Docusign\Api\SigningGroupApi($config);
$my_signing_group = new \Docusign\eSign\Model\SigningGroup();
$my_signing_group->setGroupName('Managers');
$my_signing_group->setGroupType('sharedSigningGroup'); # this value means it's a signing group
# every user must have an email address and a user name
$user_item1 = new \Docusign\eSign\Model\SigningGroupUser();
$user_item1->setEmail('sally@example.com');
$user_item2->setUserName('Sally Doe');
$user_item2 = new \Docusign\eSign\Model\SigningGroupUser();
$user_item1->setEmail('dan@example.com');
$user_item2->setUserName('Dan Dan');
$user_items = [];
array_push($user_items, $user_item1); Ā
array_push($user_items, $user_item2); Ā
mySigningGroups->setUsers($user_items);
$signing_group_info = new \Docusign\eSign\Model\SigningGroupInformation();
$groups = [];
array_push($groups, $my_signing_group); Ā
$signing_group_info->setGroups($groups);
$signing_groups_api.createList($account_id, $signing_group_info);
Python
# You would need to obtain an accessToken using your chosen auth flow
api_client = ApiClient()
api_client.host = base_path
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
signing_groups_api = SigningGroupsApi(api_client)
my_signing_group = SigningGroup()
my_signing_group.group_name = 'Managers'
my_signing_group.group_type = 'sharedSigningGroup' # this value means it's a signing group
# every user must have an email address and a user name
user_item1 = SigningGroupUser()
User_item1.email = 'sally@example.com'
user_item2.user_name = 'Sally Doe'
user_item2 = SigningGroupUser()
User_item1.email = 'dan@example.com'
user_item2.user_name = 'Dan Dan'
user_items = []
user_items.append(user_item1) Ā
user_items.append(user_item2)
my_signing_groups.users = user_items
signing_group_info = SigningGroupInformation()
groups = []
groups.append(my_signing_group)
signing_group_info.groups = groups
signing_groups_api.createList(accountId, signing_group_info)
Ruby
# You would need to obtain an accessToken using your chosen auth flow
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
signing_groups_api = DocuSign_eSign::SigningGroupsApi.new api_client
my_signing_group = DocuSign_eSign::SigningGroup.new
my_signing_group.group_name = 'Managers'
my_signing_group.group_type = 'sharedSigningGroup' # this value means it's a signing group
# every user must have an email address and a user name
user_item1 = DocuSign_eSign::SigningGroupUser.new
User_item1.email = 'sally@example.com'
user_item2.user_name = 'Sally Doe'
user_item2 = DocuSign_eSign::SigningGroupUser.new
User_item1.email = 'dan@example.com'
user_item2.user_name = 'Dan Dan'
user_items = []
user_items.push(user_item1) Ā
user_items.push(user_item2)
my_signing_groups.users = user_items
signing_group_info = DocuSign_eSign::SigningGroupInformation.new
groups = []
groups.push(my_signing_group)
signing_group_info.groups = groups
signing_groups_api.createList(account_id, signing_group_info)
Notes: The code above can only be run once. If you try to add another signing group with the same name, that wonāt result in any changes. You would need to call the Update() method to update the signing group. (You can also call the UpdateList() method to rename it). Also note thereās a maximum of 50 signing groups allowed per account.
As always, if you have any questions or suggestions about this blog series, feel free to email me.
Ā